🎮
08 动画控制器
首先在动画系统内添加 blend tree 设置好动画
随后在代码内控制速度对应的动画播放
注:注释的代码是不相关的代码,是前面的
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41
| using System; using System.Collections; using System.Collections.Generic; using UnityEngine; using UnityEngine.AI;
public class PlayerController : MonoBehaviour { private Animator anim;
private void Awake() { anim = GetComponent<Animator>(); }
private void Update() { SwitchAnimation(); }
void SwitchAnimation() { anim.SetFloat("Speed", agent.velocity.sqrMagnitude); }
}
|
09 改进细节 (shader graph)
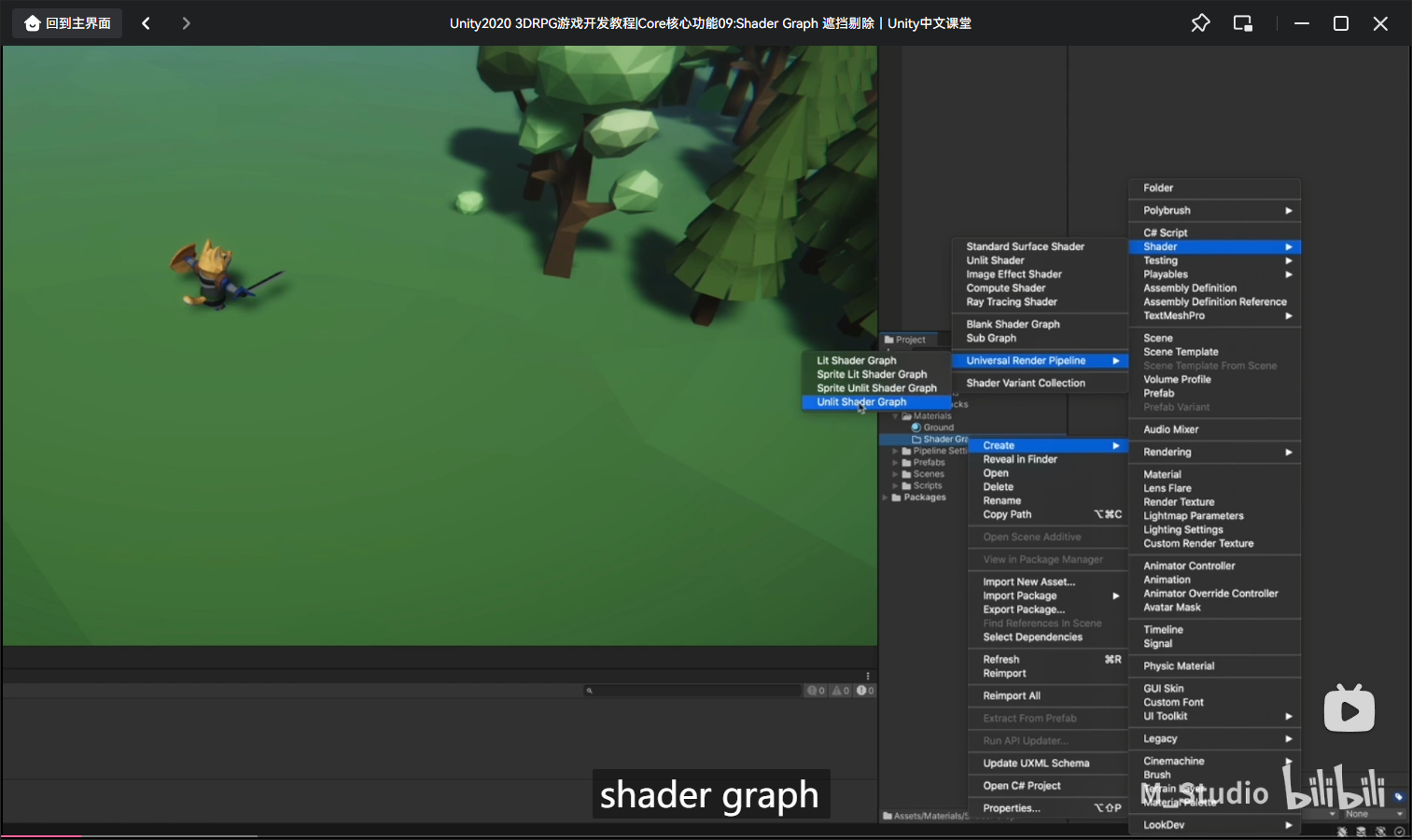
遮挡
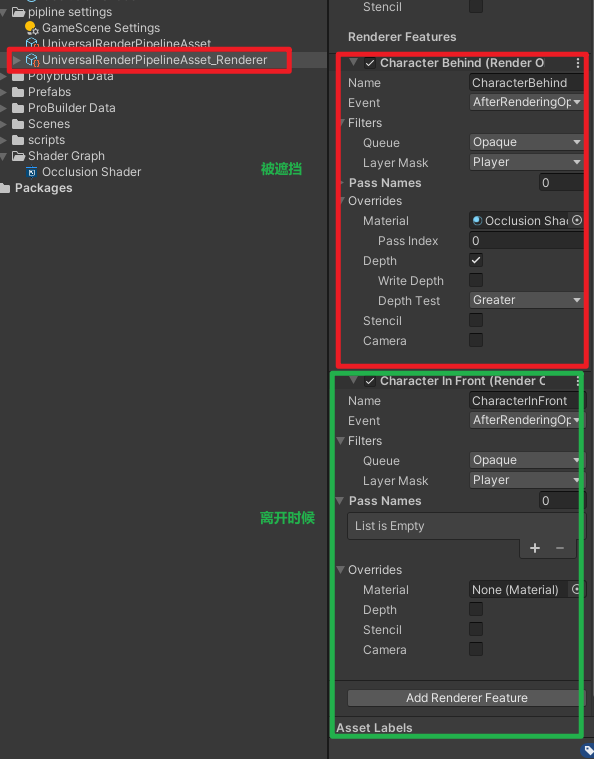
取消树的射线 改变树的图层为 🐉忽略射线🐉
第二种方法:由于代码中的碰撞是collider,只需要取消物体的mesh collider 即可
10 设置敌人
将史莱姆和蜗牛的材质都升级到urp材质
创建个脚本叫 enemy controller.cs
1 2
| [RequireComponent(typeof(NavMeshAgent))]
|
敌人的遮挡剔除
设置一个图层为enemy
回到pipline settings找到render 在layer mask中 选中enemy(那个是可以多选的),同样infront内layer mask 也要选上

修改鼠标指针贴图
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24
| void SetCursorTexture() { Ray ray = Camera.main.ScreenPointToRay(Input.mousePosition); if (Physics.Raycast(ray, out hitInfo)) { switch (hitInfo.collider.gameObject.tag) { case "Ground": Cursor.SetCursor(target,new Vector2(16,16),CursorMode.Auto); break; ----------------------- case "Enemy": Cursor.SetCursor(attack,new Vector2(16,16),CursorMode.Auto); break; ----------------------- } } }
|
😀
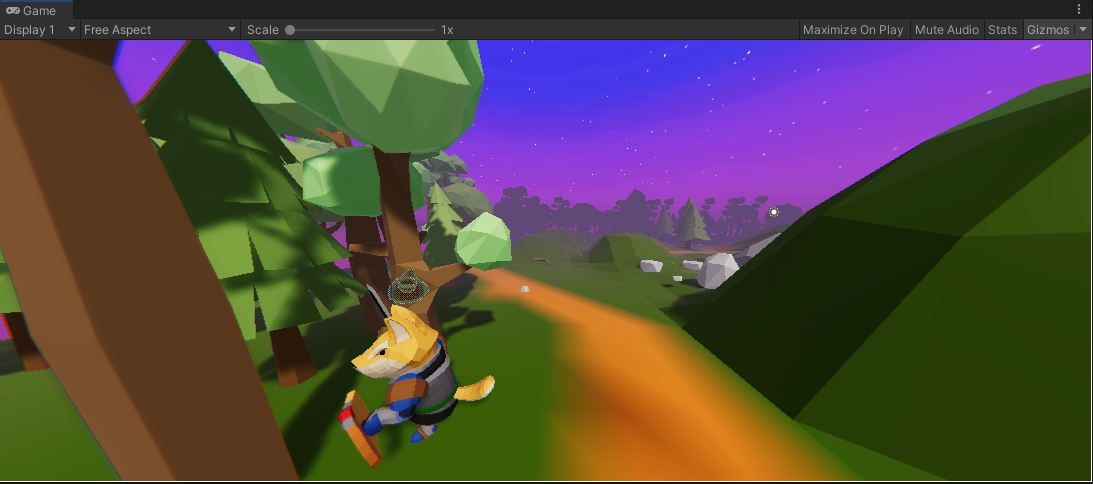
11 点击敌人玩家跑过去攻击动画
协程
//订阅事件
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81
| using System; using System.Collections; using System.Collections.Generic; using UnityEngine; using UnityEngine.AI;
public class PlayerController : MonoBehaviour { private NavMeshAgent agent; private Animator anim; private GameObject attackTarget;
private float lastAttackTime;
private void Awake() { agent = GetComponent<NavMeshAgent>(); anim = GetComponent<Animator>(); }
private void Start() { MouseManager.Instance.OnMouseClicked += MoveToTarget; MouseManager.Instance.OnEnemyClicked += EventAttack; }
private void Update() { SwitchAnimation(); lastAttackTime -= Time.deltaTime; }
void SwitchAnimation() { anim.SetFloat("Speed", agent.velocity.sqrMagnitude); }
void MoveToTarget(Vector3 target) { agent.destination = target; agent.isStopped = false;
}
private void EventAttack(GameObject target) { if (target != null) { attackTarget = target; StartCoroutine(MoveToAttackTarget()); } }
IEnumerator MoveToAttackTarget() { agent.isStopped = false; transform.LookAt(attackTarget.transform); while (Vector3.Distance(attackTarget.transform.position, transform.position) > 1) { agent.destination = attackTarget.transform.position; yield return null; } agent.isStopped = true; if (lastAttackTime<0) { anim.SetTrigger("Attack"); lastAttackTime = 0.5f; } } }
|
//获取GameObject的坐标
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75
| using System; using System.Collections; using System.Collections.Generic; using UnityEngine; using System;
public class MouseManager : MonoBehaviour { private RaycastHit hitInfo; public static MouseManager Instance; public event Action<Vector3> OnMouseClicked; public event Action<GameObject> OnEnemyClicked; public Texture2D point, doorway, attack, target, arrow;
private void Awake() { if (Instance != null) { Destroy(gameObject); }
Instance = this; }
private void Update() { SetCursorTexture(); MouseControl(); }
void SetCursorTexture() { Ray ray = Camera.main.ScreenPointToRay(Input.mousePosition); if (Physics.Raycast(ray, out hitInfo)) { switch (hitInfo.collider.gameObject.tag) { case "Ground": Cursor.SetCursor(target,new Vector2(16,16),CursorMode.Auto); break; case "Enemy": Cursor.SetCursor(attack,new Vector2(16,16),CursorMode.Auto); break; } } }
void MouseControl() { if (Input.GetMouseButtonDown(0) && hitInfo.collider != null) { if (hitInfo.collider.gameObject.CompareTag("Ground")) { OnMouseClicked?.Invoke((hitInfo.point)); } ------------------------ if (hitInfo.collider.gameObject.CompareTag("Enemy")) { OnEnemyClicked?.Invoke((hitInfo.collider.gameObject)); } ------------------------ } } }
|
//打断攻击动画
在移动方法内添加终止协程
1 2 3 4 5 6 7 8 9
| void MoveToTarget(Vector3 target) { //打断攻击动画 StopAllCoroutines(); //⭐核心⭐ agent.destination = target; agent.isStopped = false;
}
|